今回はUnity2DのRigidBodyオブジェクト移動のメモです。動く床を作成したり、壁のすり抜けを防止してみます。
はじめに
Unityのバージョンは2021.3.3f1です。
オブジェクトを動かす方法はいくつかあり、下記で何個か紹介しています。
今回は、RigidBodyをアタッチしたオブジェクトの移動についてのメモです。
実装開始
まずはアクションゲームなどでよく見る動く床を作成してみます。
動く床の作成
「2DObject」→「Sprites」→「Square」を追加。
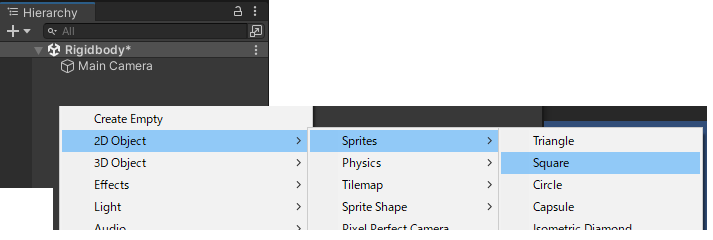
スケールを変更して横長に。Rigidbody2Dをアタッチして、BodyTypeをKinematicにします。
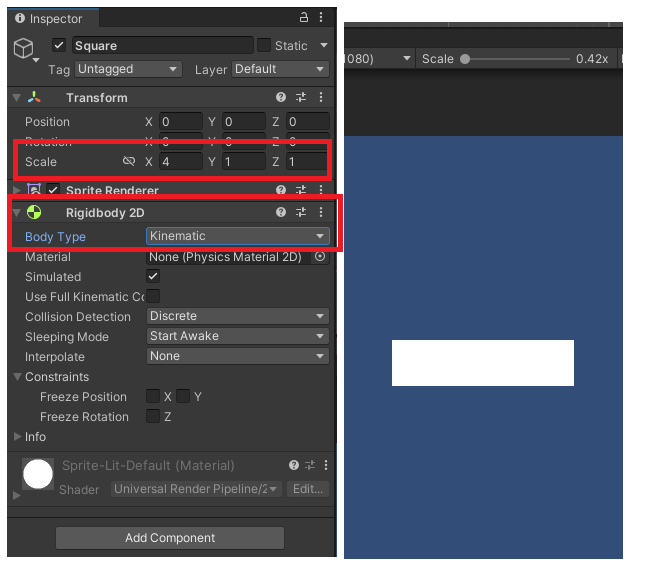
移動スクリプト
下記のスクリプトを作成します。
using UnityEngine; public class RigidBodyMove : MonoBehaviour { private Rigidbody2D _rb; void Start() { _rb = GetComponent<Rigidbody2D>(); } void FixedUpdate() { _rb.position = transform.position + transform.right * Time.deltaTime; } }
FixedUpdete内で、Rigidbodyのポジションを変更をしています。
オブジェクトにアタッチします。
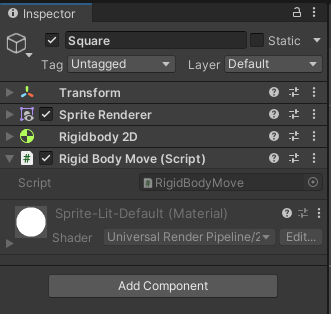
実行すると下記の様に動きます。
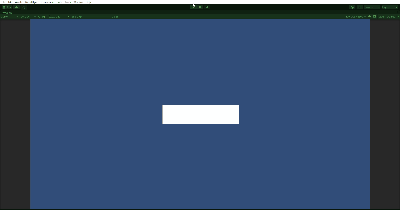
FixedUpdate内の「_rb.position」は下記の様にしても同じように動いている様に見えます。
_rb.MovePosition(transform.position + transform.right * Time.deltaTime);
他オブジェクトへの影響
次に、動く床の上にオブジェクトを乗せてみます。
動く床(Square)にBoxCollider2Dをアタッチ。
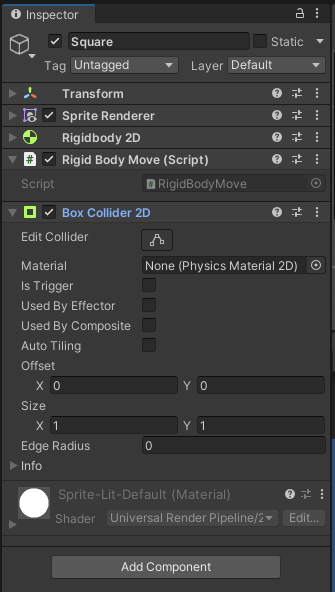
「2DObject」→「Sprites」→「Square」を追加。
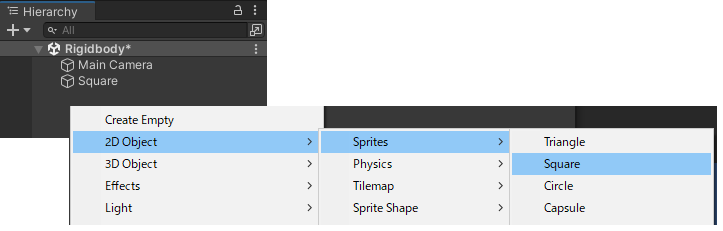
位置と色を変更して、Rigidbody2DとBoxCollider2Dをアタッチ。
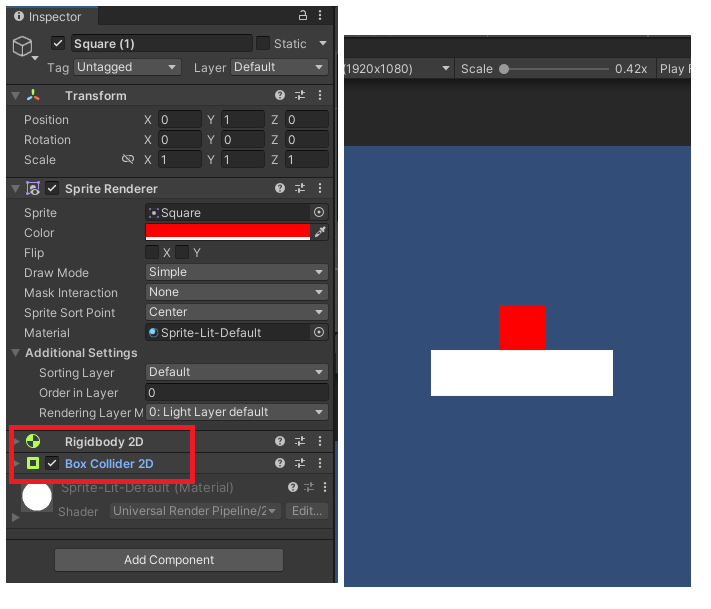
下記が「RigidBody.positon」で動かした場合です。
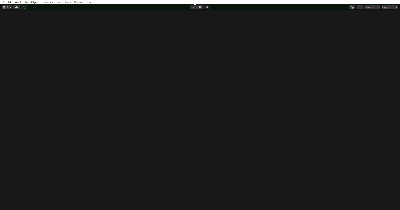
自分自身の位置だけを変更しており、他のオブジェクトには何も影響していないです。
下記が「RigidBody.MovePosition」で動かした場合です。
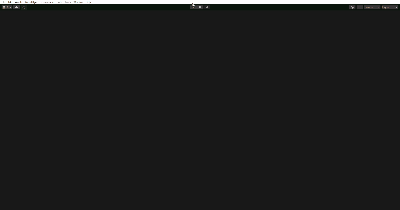
MovePositonで動かした場合、他のオブジェクトに物理的な影響を与えています。
下の方が自然な動きですが、ゲームによっては上のような動きもありそうです。
壁のすり抜け
次は壁のすり抜けについてです。
動く床のスケールを変更し、Rigidbody2DのBodyTypeをDynamicに変更、GravityScaleを0にします。
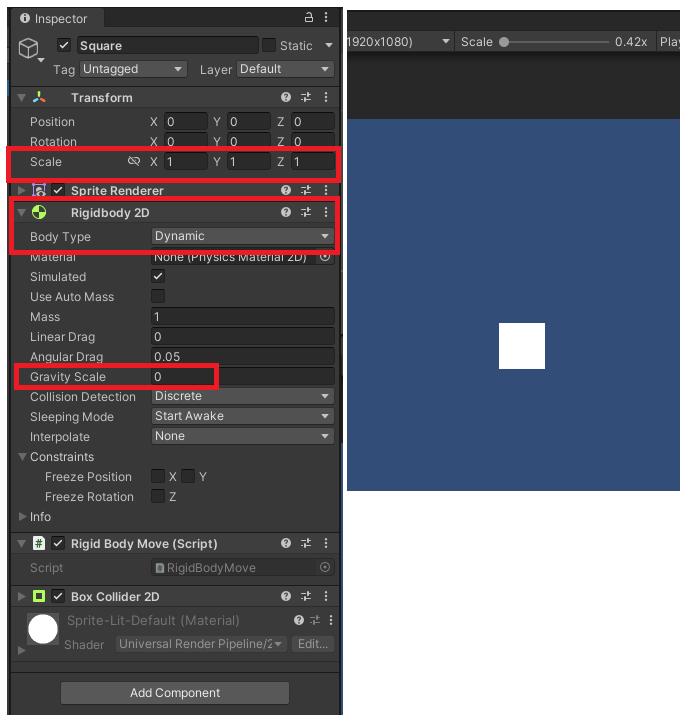
進行方向の途中に壁を設置します。
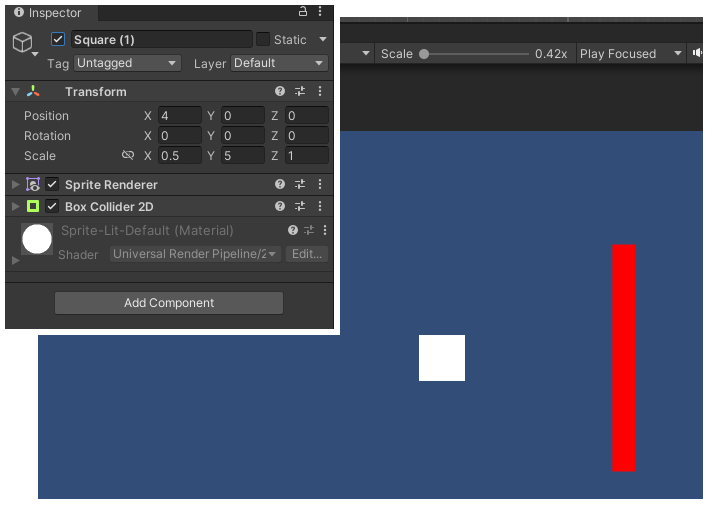
ゆっくり動く場合は壁で止まって問題ないですが、早く動く場合は壁をすり抜けたり意図しない動きとなる場合があります。下記の様な感じですね。
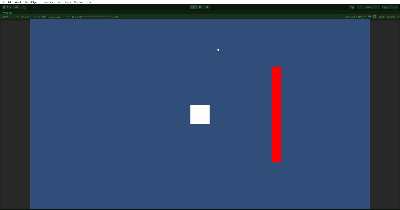
これは厳密には移動ではなく位置を変更しているので、瞬間移動的な感じとなり壁を抜けています。
すり抜けないようにするには、Rigidbody2DのCollisionDetectionを「Continuous」にすればOK。
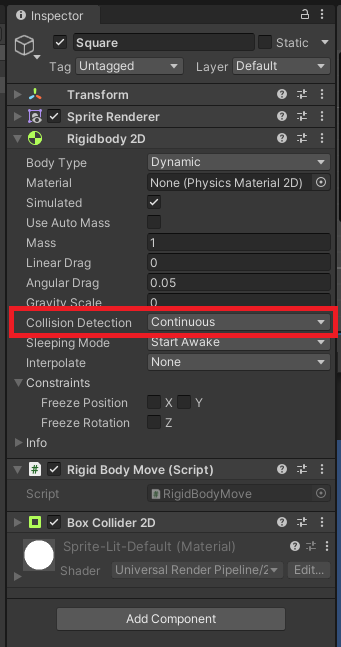
衝突検出を継続的にするので、通った道も衝突判定するという感じですね。