今回はUnityでinstantiateを利用してオブジェクトを生成した場合、指定した親オブジェクトの配下に生成されるようにする方法のメモです。
はじめに
Unityのバージョンは2021.3.3f1です。
以前、作成したシューティングゲームを元に作成していきます。Instantiateでオブジェクトを生成していればなんでもOKです。
実装開始
シューティングゲームでは、弾を打ち出すと弾のプレハブ(オブジェクト)がどんどん生成されます。
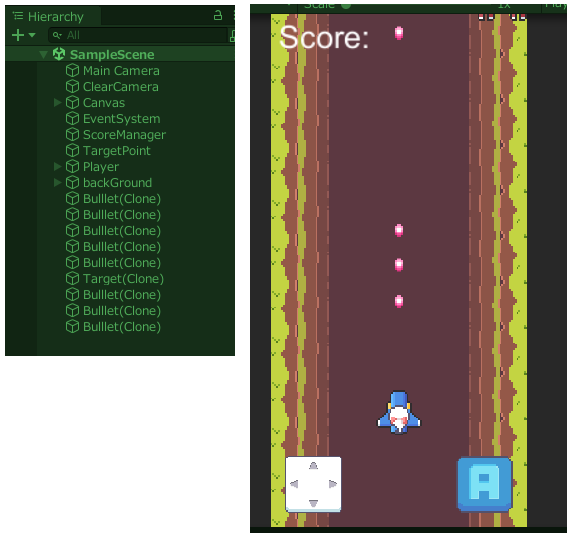
弾を打ち出しているスクリプトは下記です。Attackで弾を生成しています。
using UnityEngine; public class PlayerAttack : MonoBehaviour { [SerializeField] GameObject prefabBullet; [SerializeField] Transform bulletPoint; public void Attack() { Instantiate(prefabBullet, bulletPoint.position, bulletPoint.rotation); } }
これを下記の様にスクリプトを変更。
using UnityEngine; public class PlayerAttack : MonoBehaviour { [SerializeField] GameObject prefabBullet; [SerializeField] Transform bulletPoint; [SerializeField] GameObject _parentGameObject; public void Attack() { Instantiate(prefabBullet, bulletPoint.position, bulletPoint.rotation, _parentGameObject.transform); } }
変数に親となるオブジェクトを指定します。
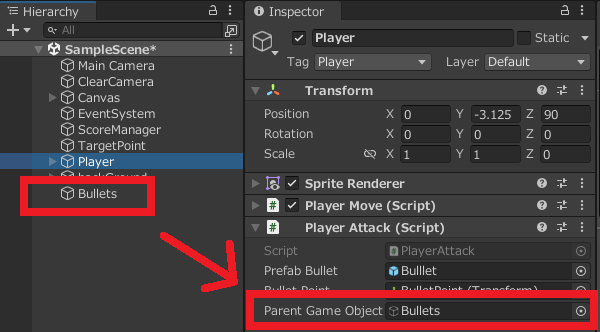
参考は下記の公式リファレンス。
実行すると、下記の様に指定されたオブジェクトの配下に弾が生成されます。
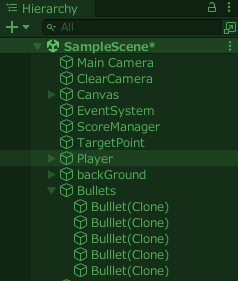
下記の様に親オブジェクトを取得する方法もあります。
GameObject.Find("Bullets"); GameObject.FindGameObjectWithTag("Bullets");
スクリプトをアタッチしているオブジェクトの配下に生成する場合は、下記の様な感じ。
using UnityEngine; public class PlayerAttack : MonoBehaviour { [SerializeField] GameObject prefabBullet; [SerializeField] Transform bulletPoint; public void Attack() { Instantiate(prefabBullet, bulletPoint.position, bulletPoint.rotation, transform); } }
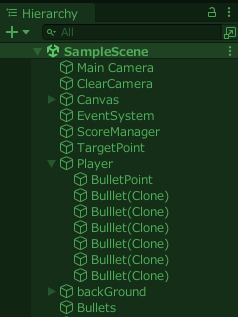