今回はUnityで「プレイヤーに向かって自動で弾を発射」するのを実装してみます。
はじめに
Unityのバージョンは2022.3.10f1です。
下記記事で「プレイヤーの侵入検知」を実装しており、途中までは似たような感じで作成していきます。「プレイヤーの位置に弾を発射」します。
実装開始
簡単なUIを作成後、スクリプトで機能を実装していきます。
UI作成
「2DObject」→「Sprites」→「Square」を2つ追加、名前を「Player」と「Ground」にします。
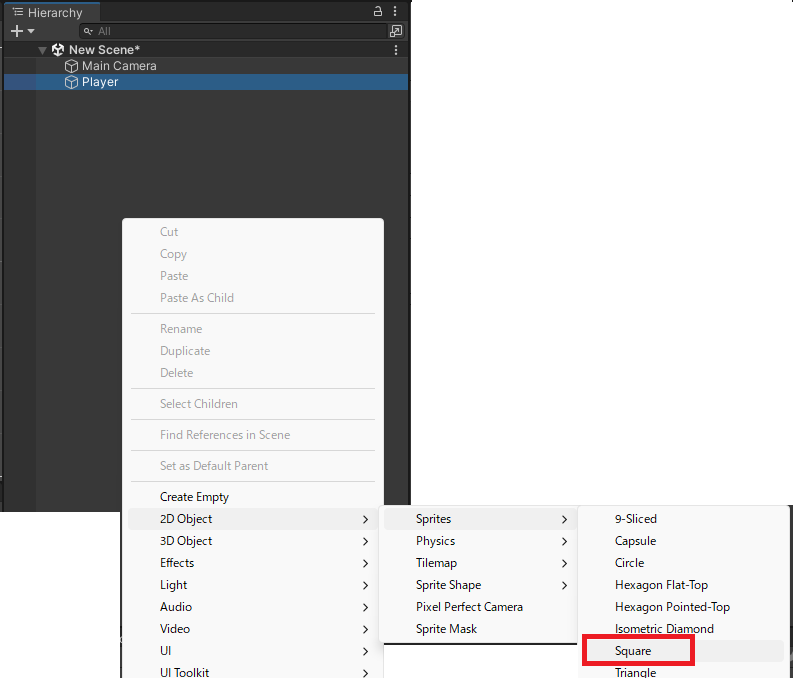
PlayerにAddComponentから「Rigidbody2D」と「BoxCollider2D」をアタッチ。
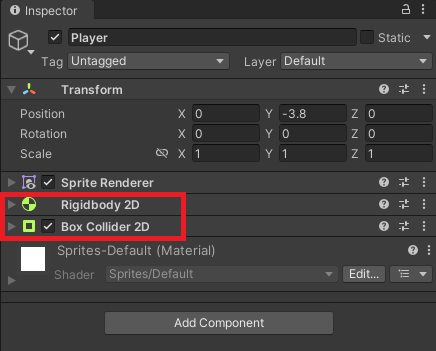
Groundには「BoxCollider2D」をアタッチ。SpriteRendererの色を変更、PositionとScaleも変更します。
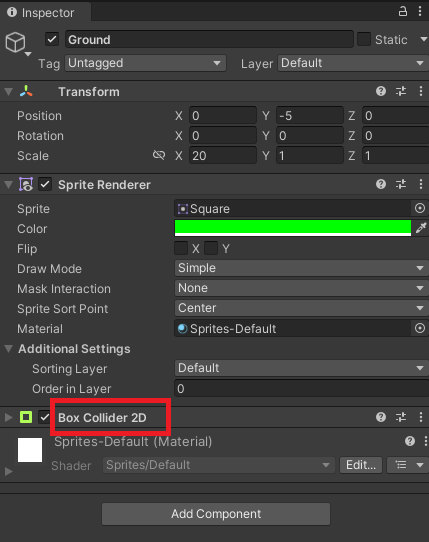
ゲーム画面が下記のような感じですね。緑色が地面で、白い四角がプレイヤーです。
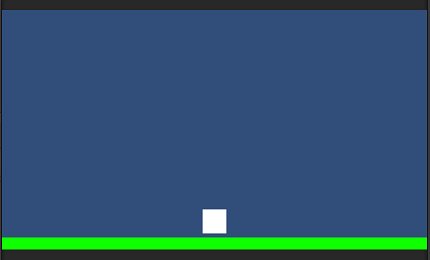
自動砲台のUI
次に弾を発射するオブジェクトを作成します、「2DObject」→「Sprites」→「Circle」を追加。名前を「Turret」とします。
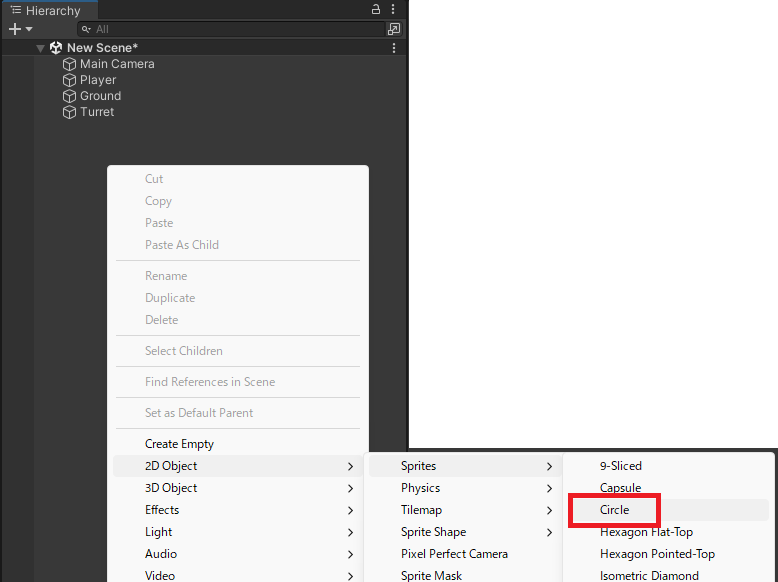
次に「2DObject」→「Sprites」→「Capsule」を追加。名前を「bullet」とします。
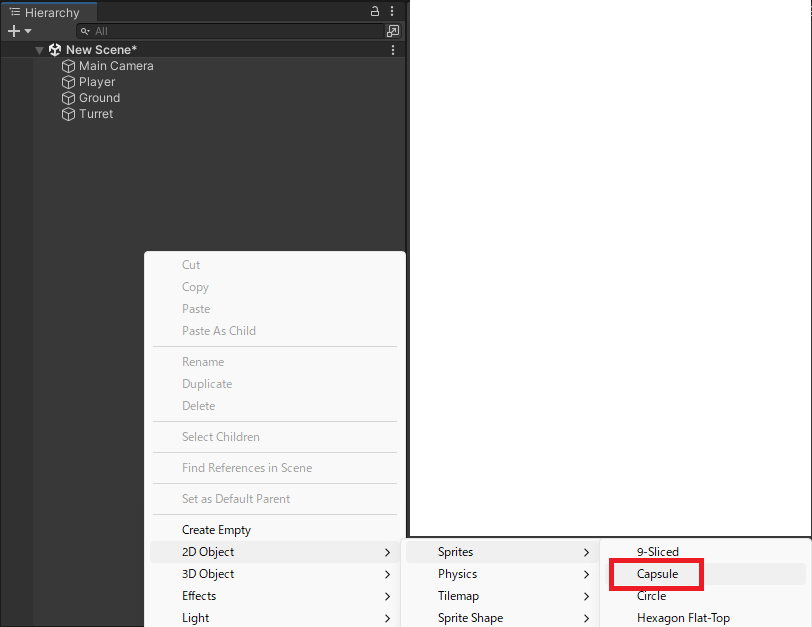
bulletのScaleを変更して、「Rigidbody2D」をアタッチします。
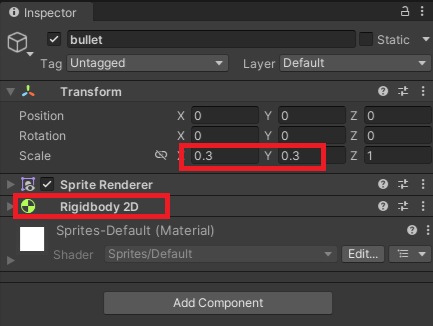
Rigidbody2DのGravityScaleは0にします。
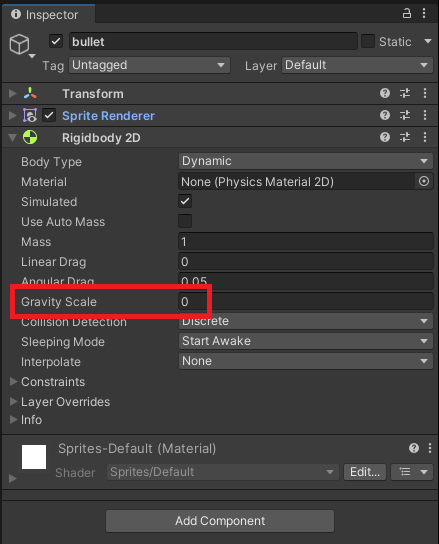
bulletをProjectフォルダに移動して、プレハブ化しておきます。Hierarchyのbulletは削除します。
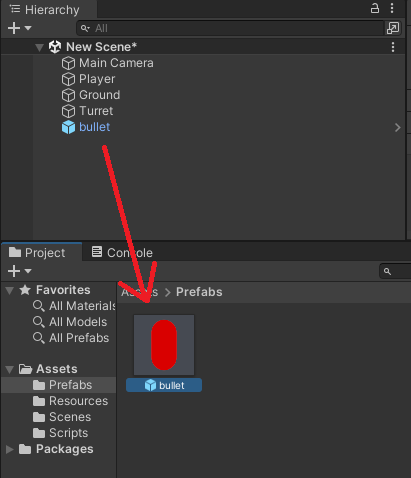
これで画面の準備は完了です。
スクリプトの作成
各スクリプトを作成していきます。まずはPlayerにアタッチするスクリプト。キー操作でプレイヤーが動くようにします。
using UnityEngine; public class Player : MonoBehaviour { private float _Speed = 5.0f; private Rigidbody2D _rb; private void Awake() { _rb = GetComponent<Rigidbody2D>(); } void Update() { float inputX = Input.GetAxisRaw("Horizontal"); _rb.velocity = new Vector2(inputX * _Speed, 0); } }
次に弾を生成するスクリプトを作成します。下記の「Turret」、「Bullet」スクリプトにて、3秒ごとにターゲットに向かって弾を生成しています。
using UnityEngine; public class Turret : MonoBehaviour { [SerializeField] private bullet _bullet; [SerializeField] private Transform targetPos; private float timer; void Update() { timer += Time.deltaTime; if (timer > 3.0f) { timer = 0f; Shoot(); } } void Shoot() { Vector3 dir = (targetPos.position - transform.position).normalized; bullet bulletIns = Instantiate(_bullet, transform); bulletIns.Init(dir); } }
using UnityEngine; public class bullet : MonoBehaviour { private Rigidbody2D _rb; private void Awake() { _rb = GetComponent<Rigidbody2D>(); } public void Init(Vector3 dir) { _rb.velocity = dir * 15f; } }
それぞれのスクリプトをオブジェクトにアタッチします。
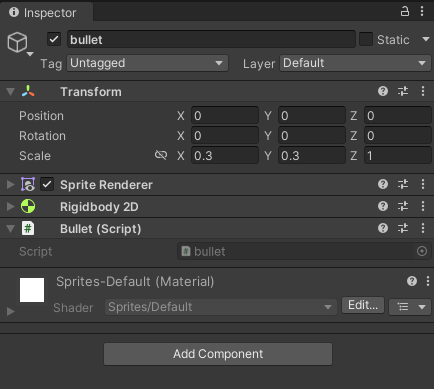
Turretは下記のような感じにパラメータをセット。
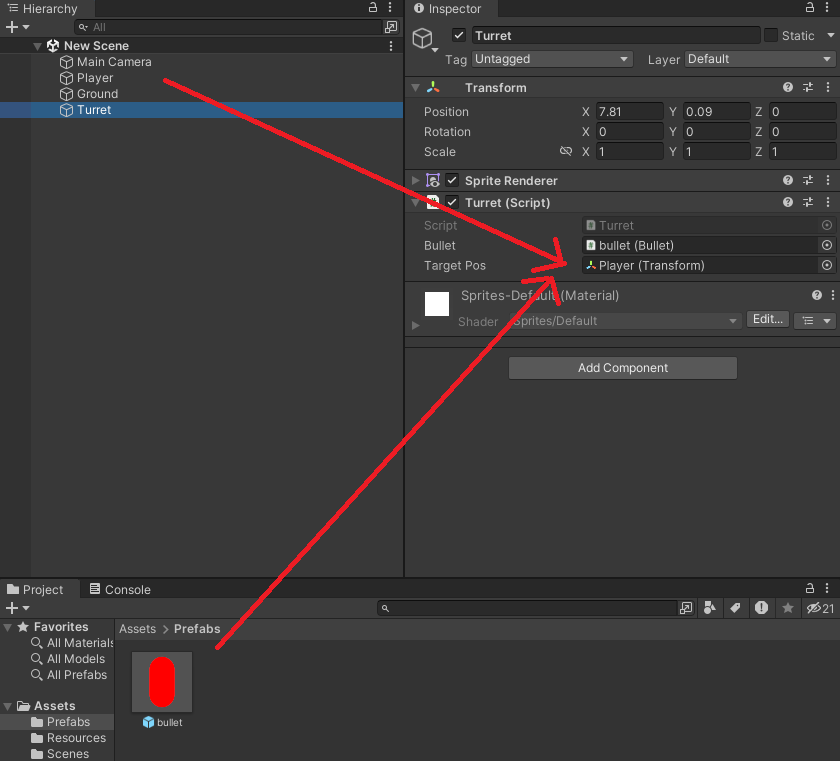
実行してみると、下記のようにプレイヤーに向かって弾が発射されますが、弾の向きがおかしいので直していきます。
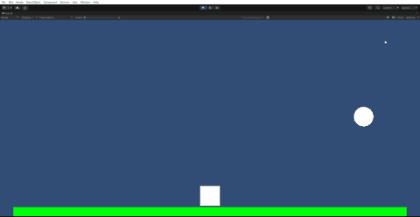
bulletのスクリプトを下記に変更します。
using UnityEngine; public class bullet : MonoBehaviour { private Rigidbody2D _rb; private void Awake() { _rb = GetComponent<Rigidbody2D>(); } public void Init(Vector3 dir) { transform.rotation = Quaternion.FromToRotation(Vector3.up, dir); _rb.velocity = dir * 15f; } }
実行すると下記のような感じに。発射時のプレイヤーの位置に向かって弾を発射します。
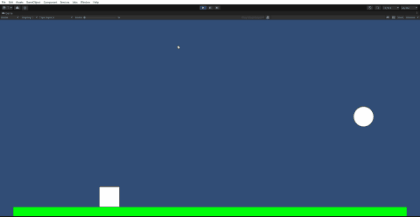