UnityのLineRendererを利用して、マウスドラッグで画面に線を描いてみます。
はじめに
Unityのバージョンは2021.3.14f1です。公式リファレンスでは「3D空間にラインを描画するために使います」とあります。
今回は、下記のように2Dで簡単に実装していきます。
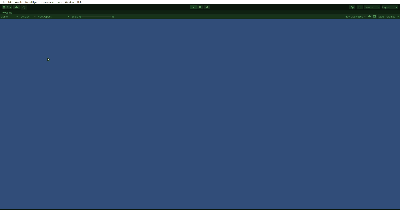
実装開始
どのように動作するかを簡単に確認して、スクリプトで実装していきます。
オブジェクトの作成
CreateEmptyで空のオブジェクトを追加。
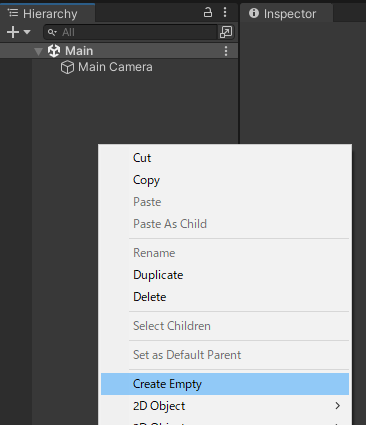
名前をLineDrawに変更、「AddComponent」からLineRendrerを追加。LineRendrerのマテリアルをSprites-Defaultに変更します。
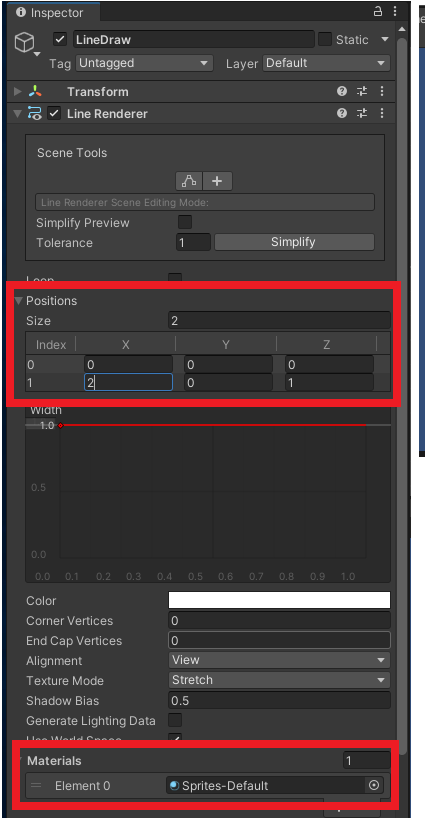
下記のようにPositionsのサイズ、座標を指定すると、画面に線を引くことが出来ます。
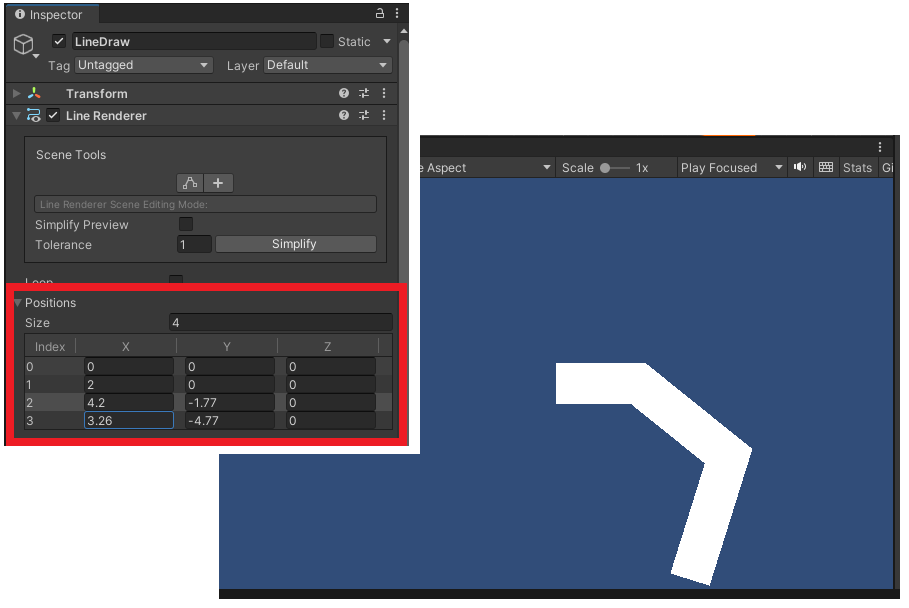
これをマウスドラッグにあわせてポイントを指定するようにスクリプトで実装していきます。
スクリプトの作成
下記のスクリプトを作成します、名前はLineDraw。
using UnityEngine; public class LineDraw : MonoBehaviour { [SerializeField] private LineRenderer _rend; [SerializeField] private Camera _cam; private void Update() { Vector2 mousePos = _cam.ScreenToWorldPoint(Input.mousePosition); if (Input.GetMouseButton(0)) SetPosition(mousePos); } private void SetPosition(Vector2 pos) { _rend.positionCount++; _rend.SetPosition(_rend.positionCount - 1, pos); } }
「Input.GetMousButton(0)」はマウスを左クリックしている時にtrueを返します。クリックしている間、マウスの位置(座標)をLineRendrerにセットします。
スクリプトをアタッチして、パラメータをセット。
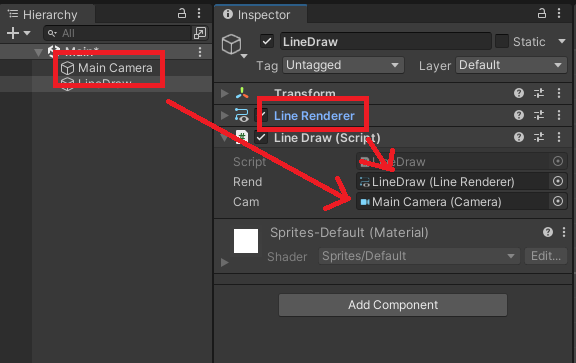
また、LineRendrerのPositionsのサイズは0にしておきます。
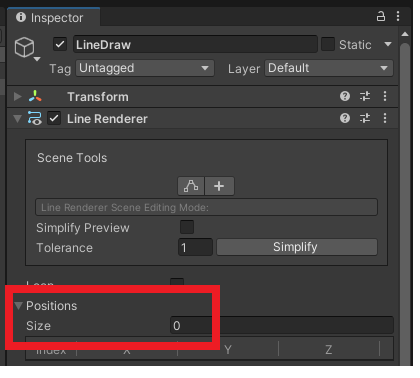
Widthで線の太さを変えたり、CornerVertices、EndCapVerticesで線に丸みをつけることができます。
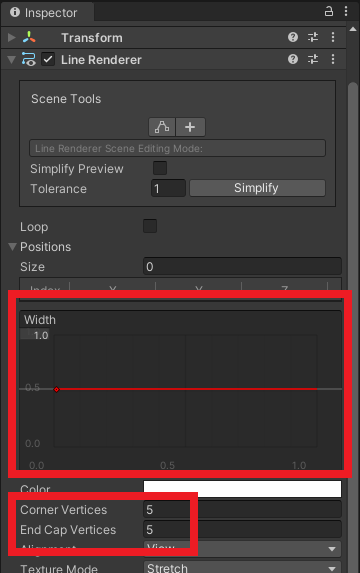
スクリプトの修正
上のスクリプトで一応動くのですが、2点修正します。一つは一度マウスを離して、もう一度マウスでなぞるとつながってしまう点。もう一つはマウスクリック中、常にポイントを作成するのでかなりのポイント数になる点です。
修正したスクリプトが下記になります。
using UnityEngine; public class LineDraw : MonoBehaviour { [SerializeField] private LineRenderer _rend; [SerializeField] private Camera _cam; private int posCount = 0; private float interval = 0.1f; private void Update() { Vector2 mousePos = _cam.ScreenToWorldPoint(Input.mousePosition); if (Input.GetMouseButton(0)) SetPosition(mousePos); else if (Input.GetMouseButtonUp(0)) posCount = 0; } private void SetPosition(Vector2 pos) { if (!PosCheck(pos)) return; posCount++; _rend.positionCount = posCount; _rend.SetPosition(posCount - 1, pos); } private bool PosCheck(Vector2 pos) { if (posCount == 0) return true; float distance = Vector2.Distance(_rend.GetPosition(posCount - 1), pos); if (distance > interval) return true; else return false; } }
「Input.GetMouseButtonUp(0)」でマウスボタンを離した時にポイントをリセットします。これで線を引く度にリセットされます。
また、前回ポイントした場所と現在位置の距離を比較して一定以上の場合のみ新たにポイントをセットするようにします。インターバルで距離を指定できますが、距離が大きすぎると線がガタガタになり、距離が小さすぎるとポイントを多く作成してしまうので適当な値にしておきます。