今回はHashSet(重複無しのリスト)を利用してみます。Unityというより少しプログラム的なメモになります。
はじめに
HashSetを簡単に言うと、重複なしのリストです。
同じ値を入れたくないリストに利用すると便利になります。
簡単なスクリプトの例
まずは下記スクリプトを作成します。
using System.Collections.Generic; using UnityEngine; public class HashSetTest : MonoBehaviour { public List<string> Fruits; private HashSet<string> HashSetcurrent = new HashSet<string>(); private void Start() { HashSetcurrent.Clear(); HashSetcurrent.Add(Fruits[0]); foreach (var item in HashSetcurrent) { Debug.Log(item); } } }
スクリプトをオブジェクトに追加します。今回はカメラに追加しました。リスト型の「Fruits」には好きな果物を指定します。
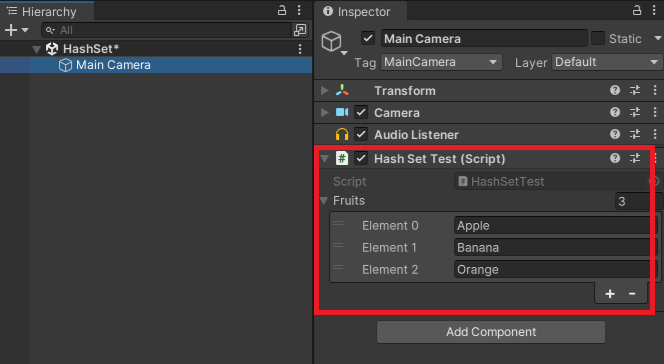
実行すると、「HashSetcurrent」に0番目の果物(リンゴ)が追加されます。
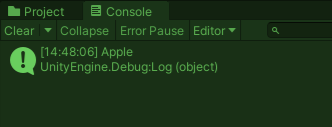
スクリプトのStartを下記に変更します。
private void Start() { HashSetcurrent.Clear(); HashSetcurrent.Add(Fruits[0]); HashSetcurrent.Add(Fruits[0]); HashSetcurrent.Add(Fruits[2]); foreach (var item in HashSetcurrent) { Debug.Log(item); } }
0番目の果物(リンゴ)を2回追加していますが、1回しか追加されません。(重複が除外されるため)
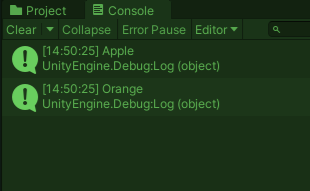
通常のListを使った場合、重複したくない場合はチェックが必要になるので、簡単になりますね。
他のメソッドを確認
主なメソッドを記載したのが下記のスクリプトです。
using System.Collections.Generic; using UnityEngine; public class HashSetTest : MonoBehaviour { public List<string> Fruits; private HashSet<string> HashSetcurrent = new HashSet<string>(); private void Start() { // 全て削除 HashSetcurrent.Clear(); // 追加 HashSetcurrent.Add(Fruits[0]); HashSetcurrent.Add(Fruits[0]); HashSetcurrent.Add(Fruits[2]); // 削除 HashSetcurrent.Remove(Fruits[0]); // 要素数を取得 Debug.Log(HashSetcurrent.Count); // 指定要素があるか確認 Debug.Log(HashSetcurrent.Contains(Fruits[1])); } }
実行すると下記のような感じになります。
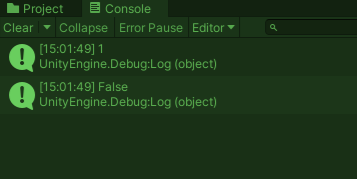