簡単な3D迷路ミニゲーム作成の第2回目を進めていきます。今回は迷路内を動くプレイヤーを設定していきます。
はじめに
前回の続きの第2回目です。第1回目は下記から。
ゲーム作成開始
では、地面と壁を設置した続きから進めていきます。まずは迷路内を動くプレイヤーを作成。
プレイヤーの作成
ヒエラルキーメニューから「3Dオブジェクト」→「スフィア」を選択。
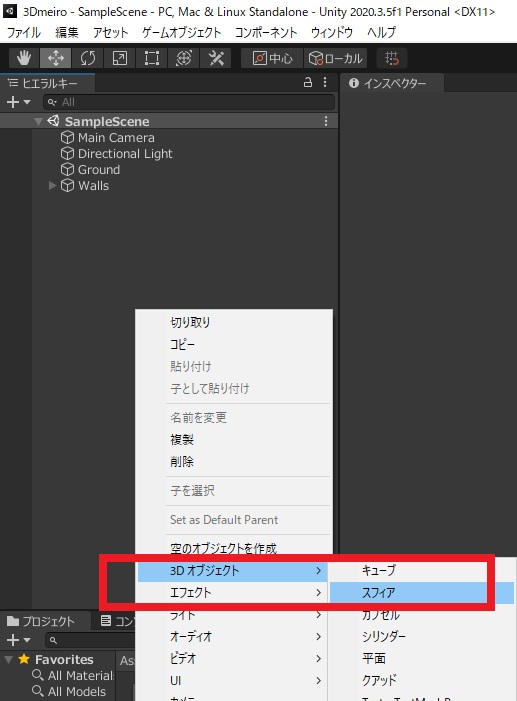
名前をPlayerに変更して、位置Xを9、Yを0.5、Zを9。スケールを全部1に変更。
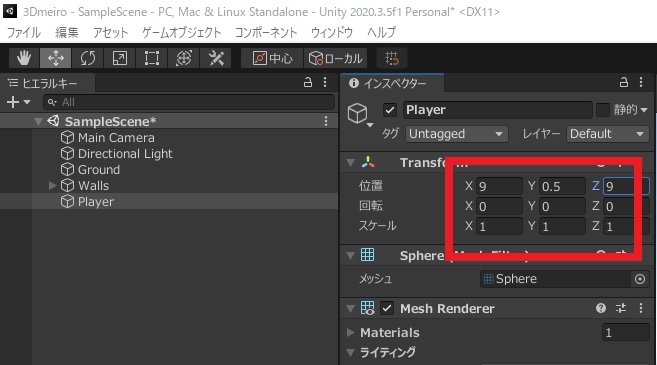
ただの丸いオブジェクトですが、一旦これを動かすプレイヤーとします。
動かせるようにスクリプト作成
Playerのコンポーネントを追加から「新しいスクリプト」を選択。名前は「PlayerMove」に。
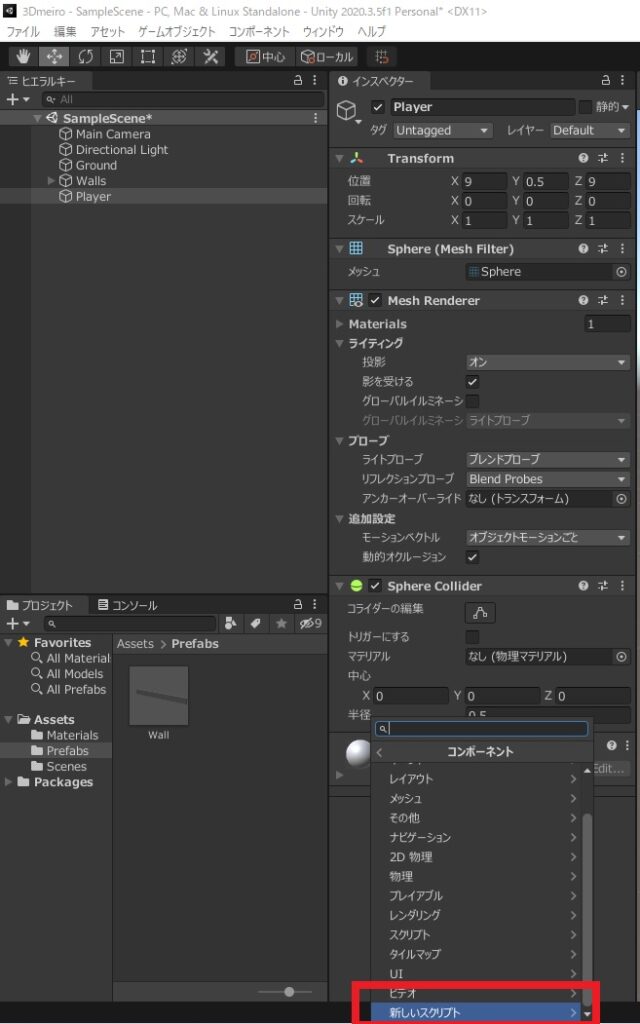
Assets内に「Scripts」フォルダを作成してその中にPlayerMoveスクリプトを移動。
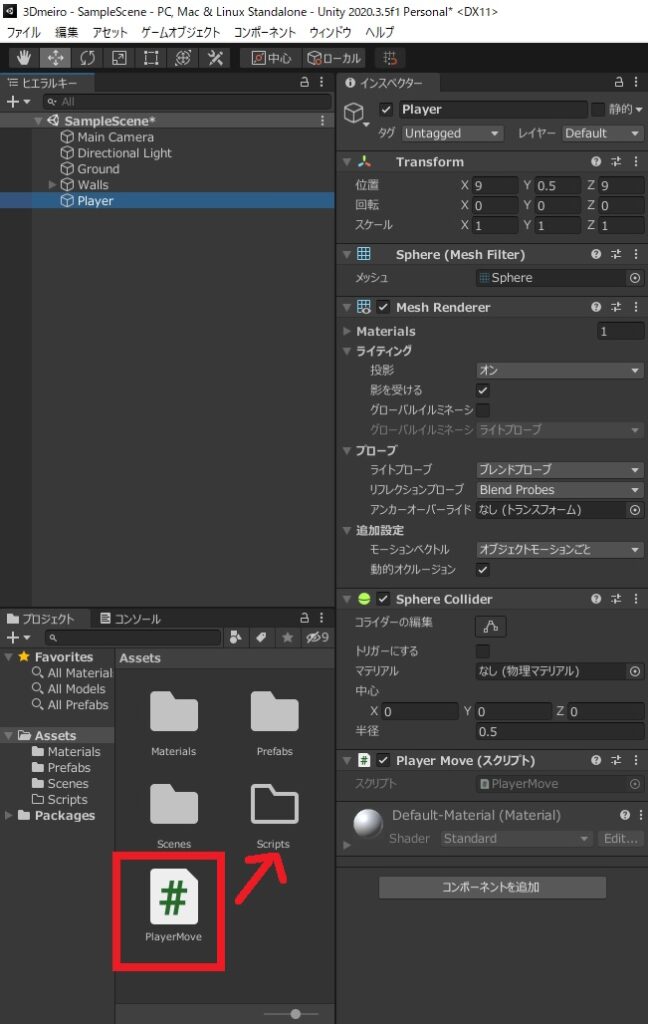
プレイやを動かすスクリプトですが、様々な方法がありますが、今回はUnityのスクリプトリファレンスにある「CharacterController.Move」を利用していきます。
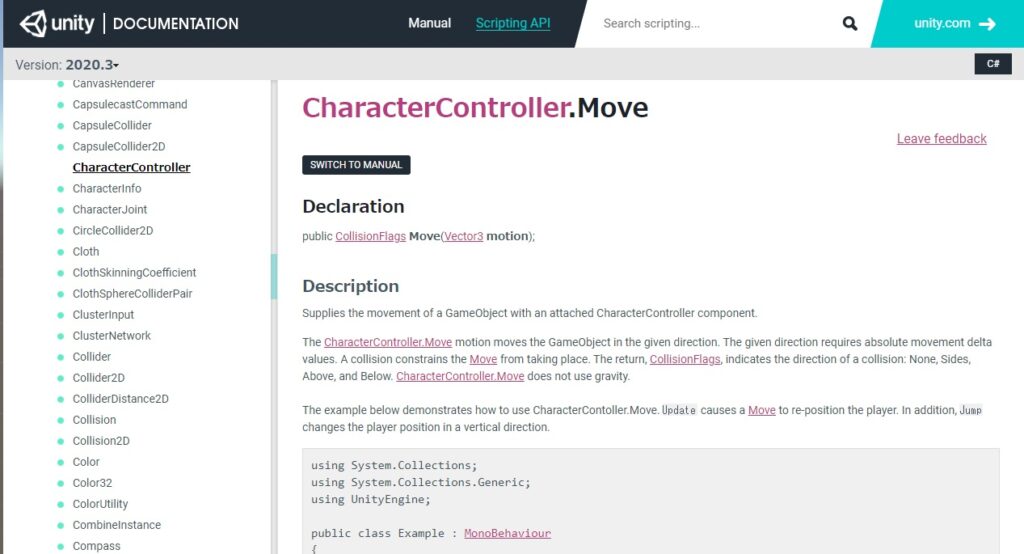
ここに記載のあるコードを丸っとコピーして貼り付け、Exampleと記載のある個所をPlayerMoveに変更、下記の様にします。
using System.Collections; using System.Collections.Generic; using UnityEngine; public class PlayerMove : MonoBehaviour { private CharacterController controller; private Vector3 playerVelocity; private bool groundedPlayer; private float playerSpeed = 2.0f; private float jumpHeight = 1.0f; private float gravityValue = -9.81f; private void Start() { controller = gameObject.AddComponent<CharacterController>(); } void Update() { groundedPlayer = controller.isGrounded; if (groundedPlayer && playerVelocity.y < 0) { playerVelocity.y = 0f; } Vector3 move = new Vector3(Input.GetAxis("Horizontal"), 0, Input.GetAxis("Vertical")); controller.Move(move * Time.deltaTime * playerSpeed); if (move != Vector3.zero) { gameObject.transform.forward = move; } // Changes the height position of the player.. if (Input.GetButtonDown("Jump") && groundedPlayer) { playerVelocity.y += Mathf.Sqrt(jumpHeight * -3.0f * gravityValue); } playerVelocity.y += gravityValue * Time.deltaTime; controller.Move(playerVelocity * Time.deltaTime); } }
一度、この状態で動かしてみます。
カメラ位置の変更
試しに動かしてみますが、カメラ位置が悪くて動いているのか分からないのでMainCameraの位置を変更。位置Xを0,Yを15,Zを-15。回転Xを45,Yを0,Zを0にします。
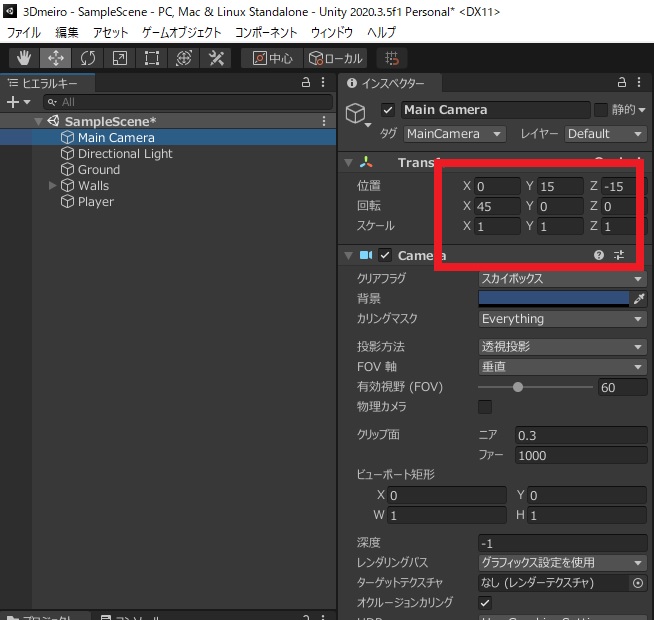
ゲーム画面が下記のように表示されていればOK。試しに実行してキーボードのWASDや矢印キーでボールが動くのを確認します。
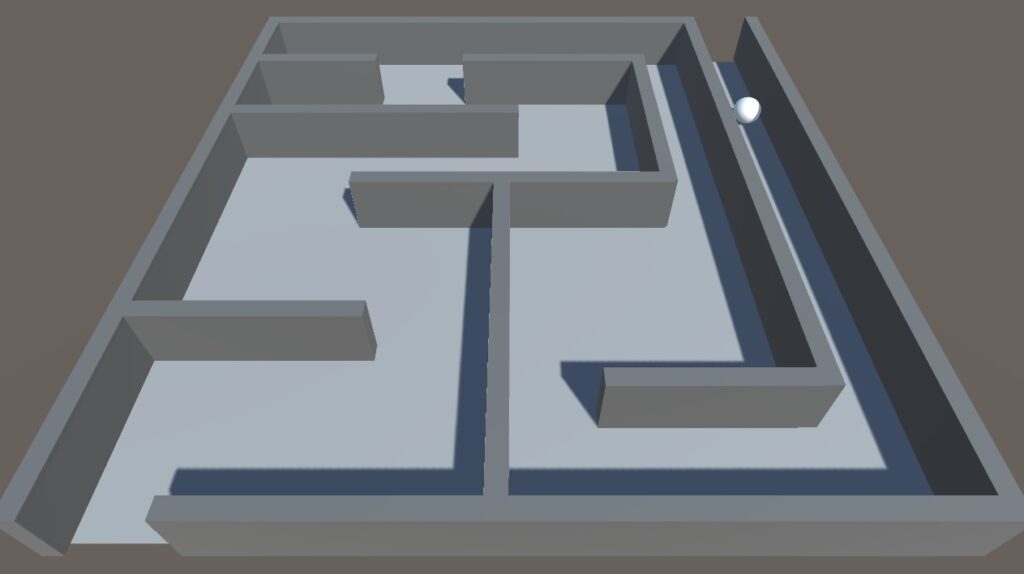
サンプルコードにはジャンプもありますが今回は不要なので削除。また、速度を簡単に変更できるようにPlayerSpeedをPublicに変更。下記のようにしておきます。
using System.Collections; using System.Collections.Generic; using UnityEngine; public class PlayerMove : MonoBehaviour { private CharacterController controller; private Vector3 playerVelocity; private bool groundedPlayer; public float playerSpeed; private void Start() { controller = gameObject.AddComponent<CharacterController>(); } void Update() { groundedPlayer = controller.isGrounded; if (groundedPlayer && playerVelocity.y < 0) { playerVelocity.y = 0f; } Vector3 move = new Vector3(Input.GetAxis("Horizontal"), 0, Input.GetAxis("Vertical")); controller.Move(move * Time.deltaTime * playerSpeed); if (move != Vector3.zero) { gameObject.transform.forward = move; } } }
PlayerのSpeedを良い感じに変更。
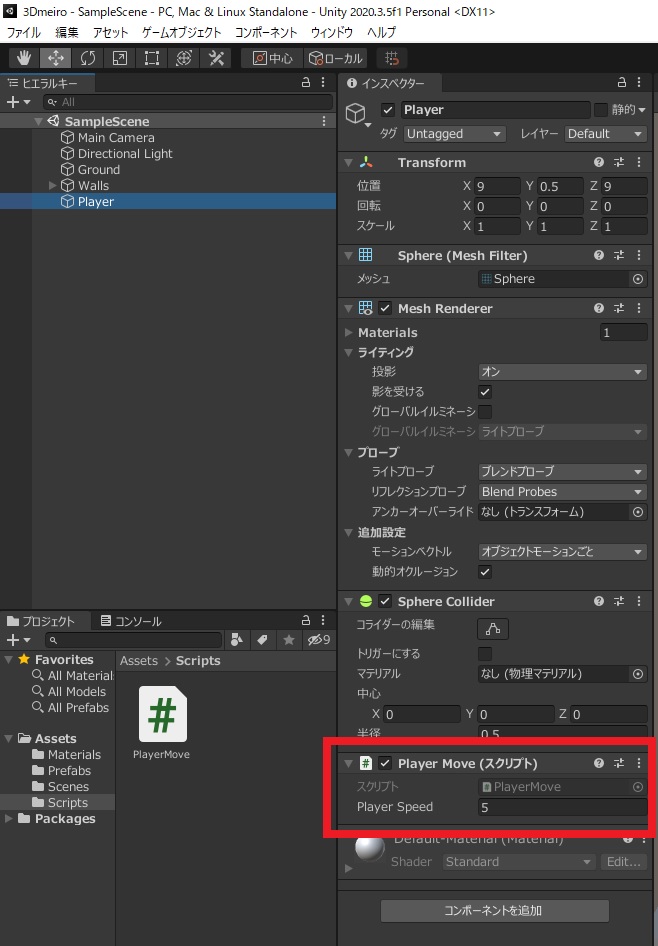
とりあえず、これで迷路の中を動くプレイヤーが完成。