今回はUnityでDOTween(HOTween v2)を利用して、画像を動かしたりフェードイン・フェードアウトをしてみます。
はじめに
Unityのバージョンは2021.3.14f1です。
ゲームクリア時に出てくる下記の様なものを作成していきます。
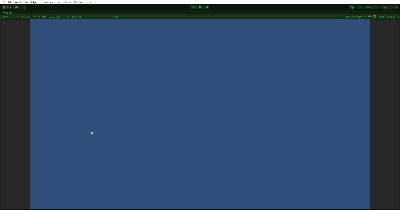
DOTweenの追加は下記にて記載しています。
画像アセットの追加
まずはUnityAssetStoreにアクセスして、2DCasualUIHDをマイアセットに追加します。
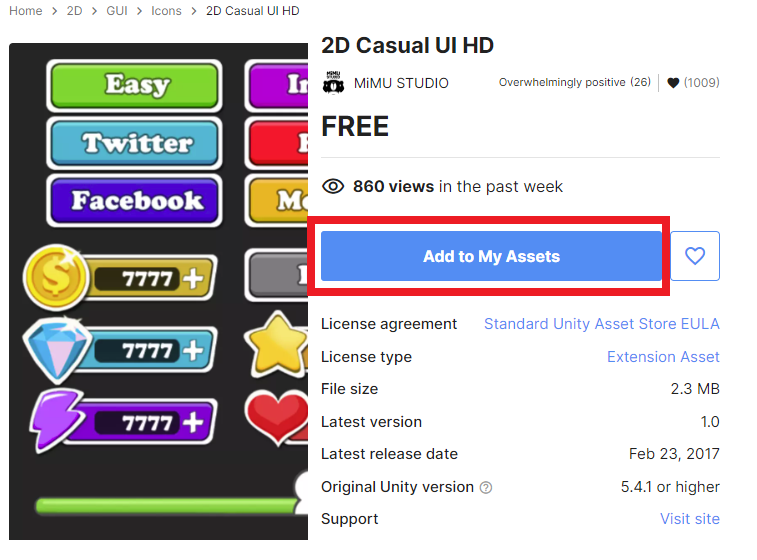
PackageManagerからDownloadをします。
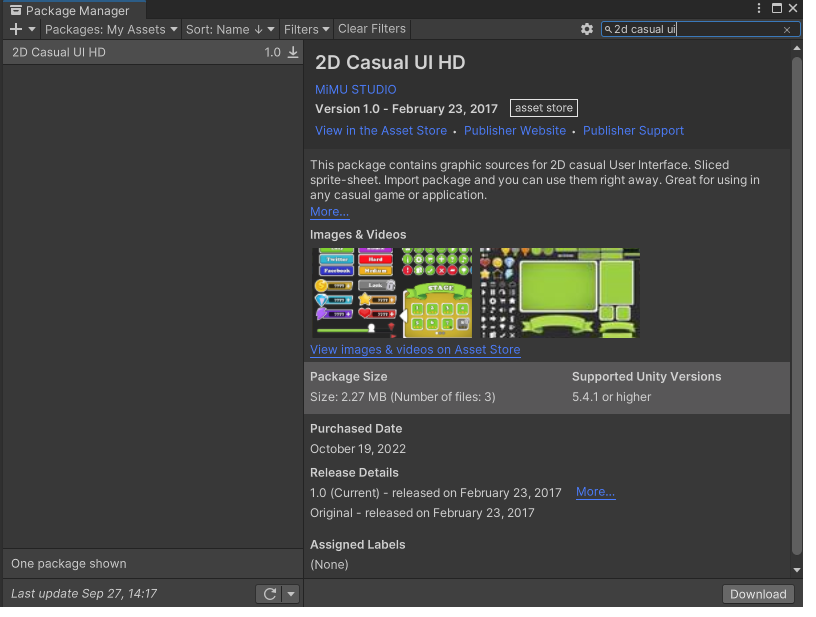
Download後に全てをimport。
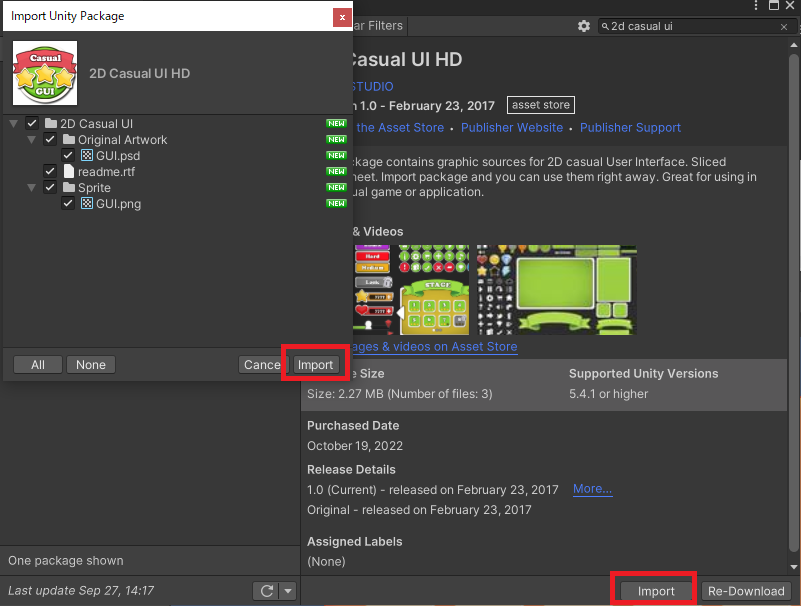
これで準備完了です。
実装開始
ここから実際に作成していきます。簡単にUIを作成した後、スクリプトで実装していきます。
UIの作成
Hierarchyで右クリックして、「UI」→「Image」を追加。
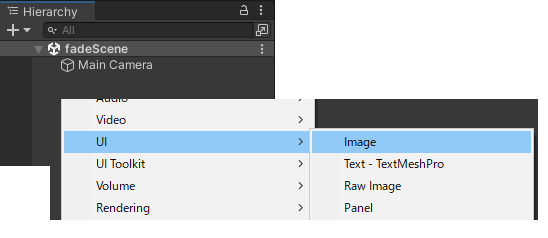
名前をPanelに変更して、幅と高さ、SourceImageを変更して下記の様にします。
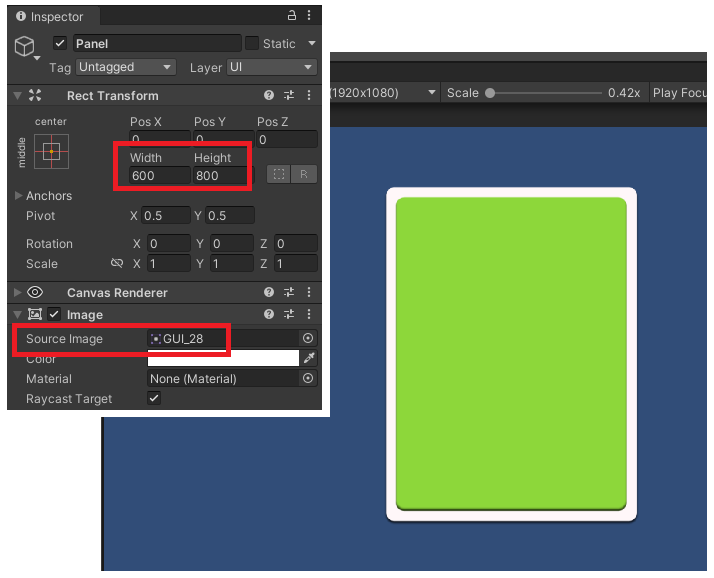
Panel配下で「UI」→「Image」を追加
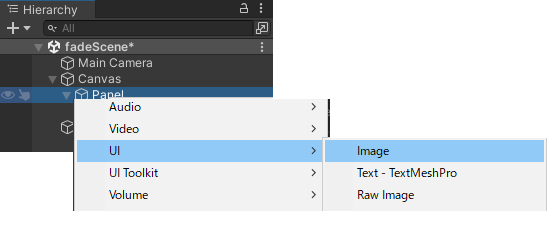
名前をPanelTitleにして位置や幅、高さを変更、SourceImageとColorを変更。下記の様にします。
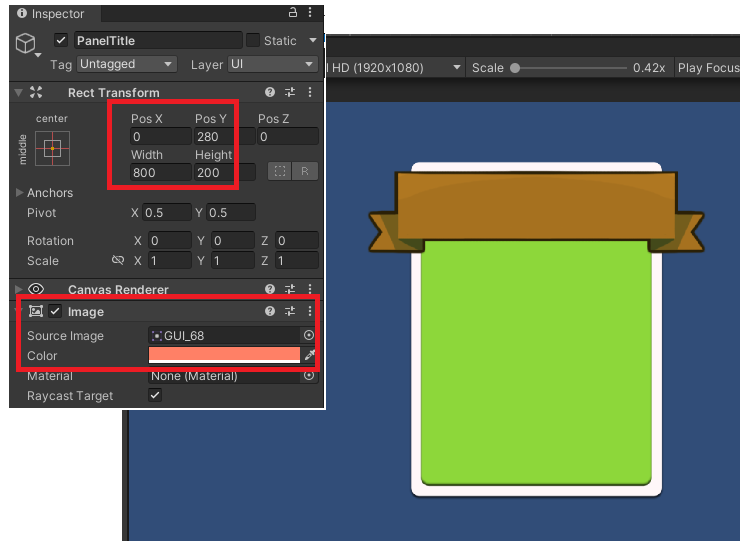
PanelTitleの配下に「UI」→「Text-TextMeshPro」を追加。
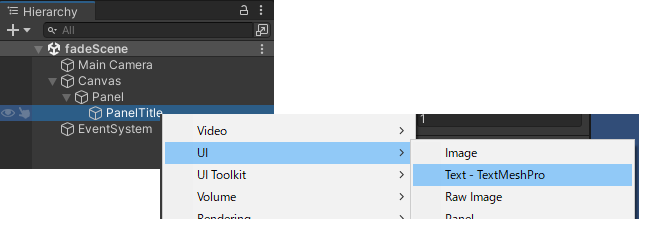
テキストを変更して下記の様にします。
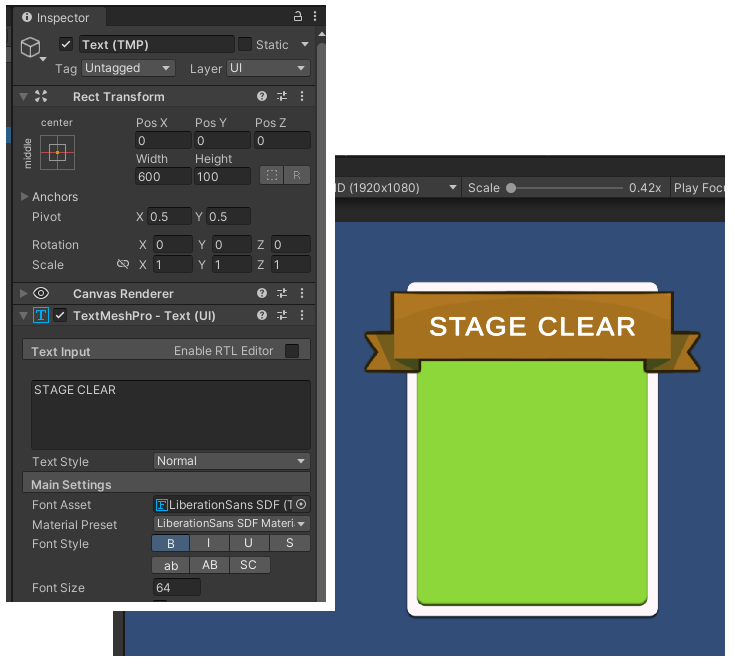
「Panel」の配下に「UI」→「Canvas」を追加。
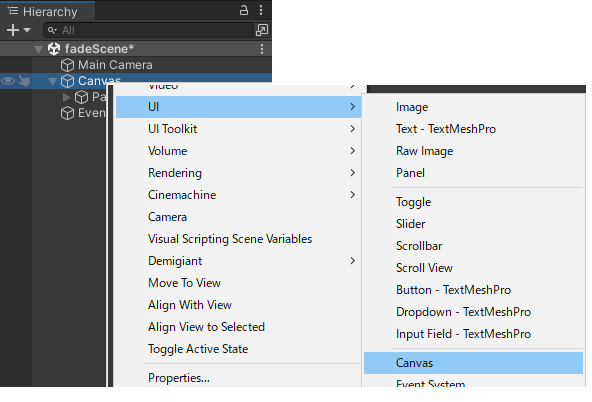
名前をStarsに変更し、更にその配下に「UI」→「Image」を追加
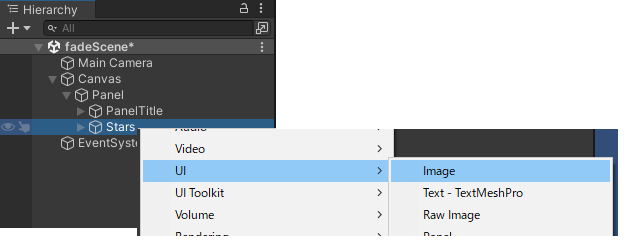
SourceImageを変更して下記の様に配置。
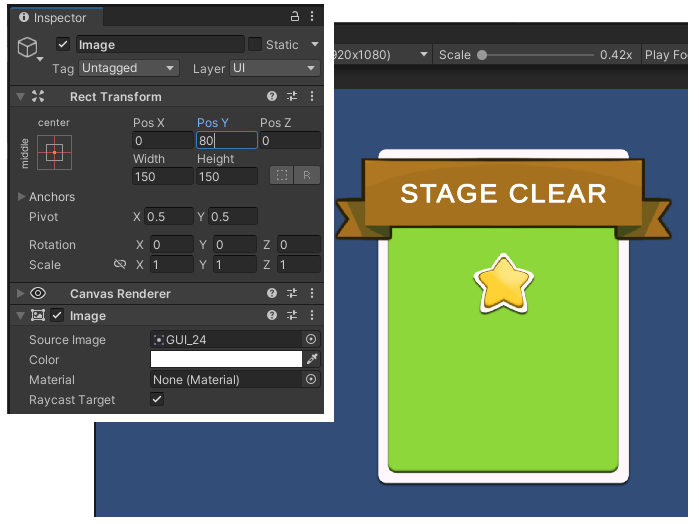
コピーして3つ、下記の様に配置します。
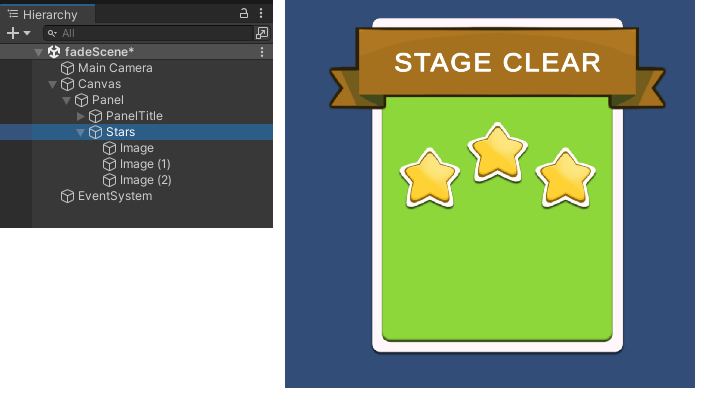
これでとりあえず簡単なUIの完成。これをDOTweenを利用して動かしてみます。
スクリプトの作成
まずは下記スクリプトを作成。DOTweenを使わず、パネルを有効/無効を切り替えてみます。
using UnityEngine; public class fade : MonoBehaviour { [SerializeField] private GameObject Panel; private void Update() { if (Input.GetKey(KeyCode.Space)) Panel.SetActive(true); if (Input.GetKey(KeyCode.A)) Panel.SetActive(false); } }
パラメータをセット
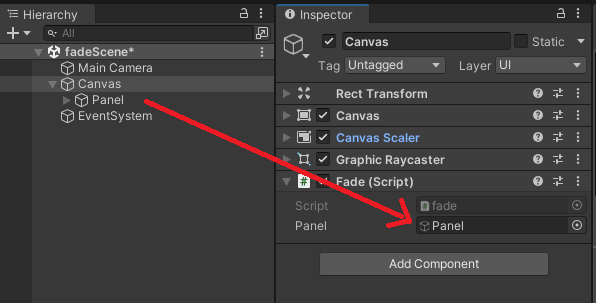
実行すると下記の様な感じでスペースキーを押すとパネルが有効になります。「パッ」と出てくる感じですね。これをDOTweenを使って動きを付けてみます。
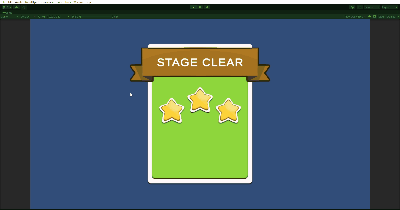
スクリプトを下記に変更。
using UnityEngine; using DG.Tweening; public class fade : MonoBehaviour { [SerializeField] private RectTransform rectTransform; private float fadeTime = 0.6f; private float fadePos = 1000f; private void Update() { if (Input.GetKey(KeyCode.Space)) FadeIn(); if (Input.GetKey(KeyCode.A)) FadeOut(); } private void FadeIn() { rectTransform.transform.localPosition = new Vector3(0f, fadePos, 0f); rectTransform.DOAnchorPos(new Vector2(0f, 0f), fadeTime, false); } private void FadeOut() { rectTransform.transform.localPosition = new Vector3(0f, 0f, 0f); rectTransform.DOAnchorPos(new Vector2(0f, fadePos), fadeTime, false); } }
実行すると下記の様な感じ、上からパネルが出てくる動きになります。
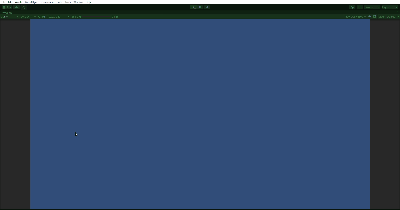
フェードイン・フェードアウト
PanelにCanvasGroupをアタッチ。
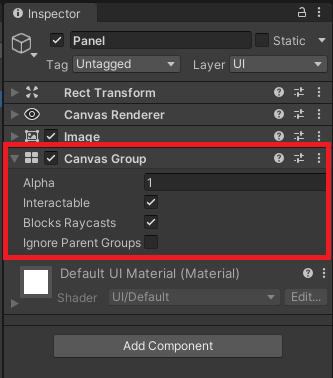
スクリプトを下記に変更。
using System.Collections; using System.Collections.Generic; using UnityEngine; using DG.Tweening; public class fade : MonoBehaviour { [SerializeField] private CanvasGroup canvasGroup; [SerializeField] private float fadeTime; private float fadePos = 1000f; private void Update() { if (Input.GetKey(KeyCode.Space)) FadeIn(); if (Input.GetKey(KeyCode.A)) FadeOut(); } private void FadeIn() { canvasGroup.alpha = 0f; canvasGroup.DOFade(1, fadeTime); } private void FadeOut() { canvasGroup.alpha = 1f; canvasGroup.DOFade(0, fadeTime); } }
パラメータをセットします。
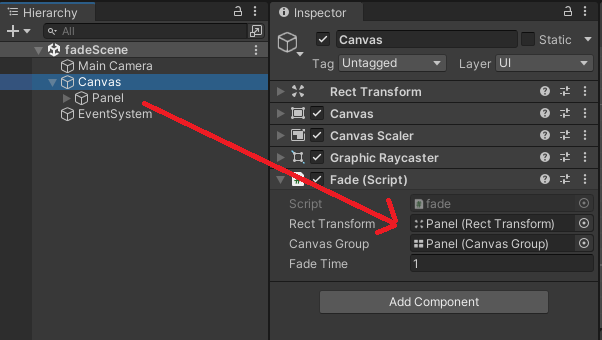
実行すると下記の様な感じに。
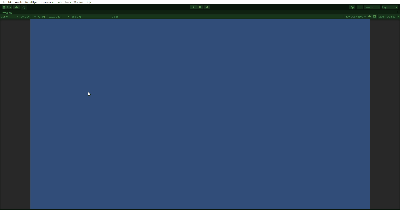