今回はUnityで「RequireComponentを使って、Rigidbodyを自動追加」するメモです。
Rigidbodyに限らずスクリプトやAnimatorなどのコンポーネントも同様に利用できます。
どんな時に使えるのか
例えば、PlayerオブジェクトにRigidbodyをアタッチし忘れてスクリプトで操作すると、下記エラーが出てきます。
MissingComponentException: There is no 'Rigidbody' attached to the "Player" game object, but a script is trying to access it. You probably need to add a Rigidbody to the game object "Player". Or your script needs to check if the component is attached before using it.
Rigidbodyをつけ忘れたな。ってことでAddComponentからRigidbodyを追加すれば解決するのですが、多くのオブジェクトにアタッチ予定のスクリプトの場合、Rigidbodyを忘れがちになる事も。
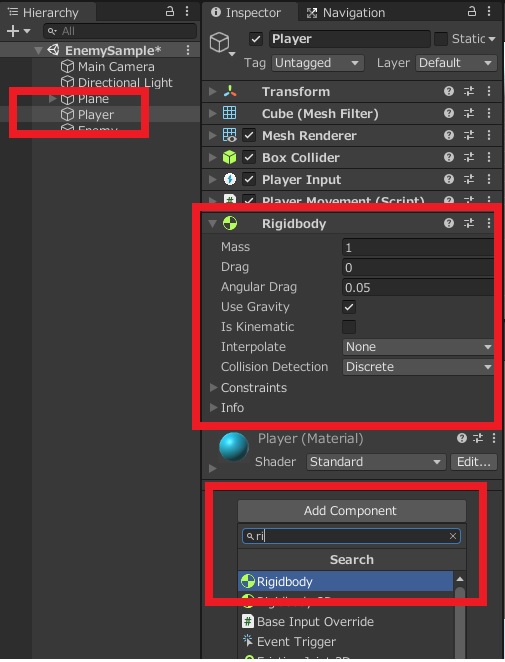
RequireComponent
そんな時は下記の様にスクリプトに「RequireComponent(typeof(Rigidbody))」を記載しておきます。
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.InputSystem; [RequireComponent(typeof(Rigidbody))] public class PlayerMovement : MonoBehaviour { [SerializeField] private float PlayerSpeed; private Rigidbody _rigidbody; private Vector3 movement; void Start() { _rigidbody = GetComponent<Rigidbody>(); } void OnMove(InputValue movementValue) { Vector2 movementVector = movementValue.Get<Vector2>(); movement = new Vector3(movementVector.x, 0.0f, movementVector.y); } void Update() { _rigidbody.velocity = movement * PlayerSpeed; } }
すると、Rigidbodyをアタッチ忘れたオブジェクトに自動で作成してくれます。下記の様にメッセージで教えてくれます。
Creating missing Rigidbody component for PlayerMovement in Player.
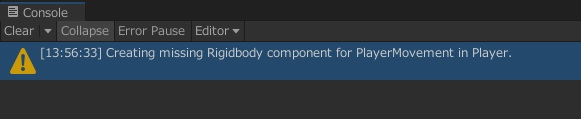
また、スクリプトをアタッチした状態でRigidbodyコンポーネントをRemove(削除)しようとすると下記のメッセージが出て削除ができなくなり、ミスで削除防止にもなります。
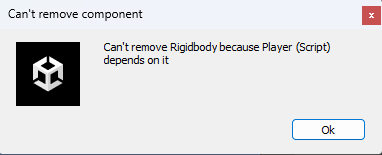
スクリプト内でプロパティの設定をしてもOKなので設定忘れも防止できるかも。
void Start() { _rigidbody = GetComponent<Rigidbody>(); _rigidbody.constraints = RigidbodyConstraints.FreezeRotation; }
下のような感じで自動で作成されます。
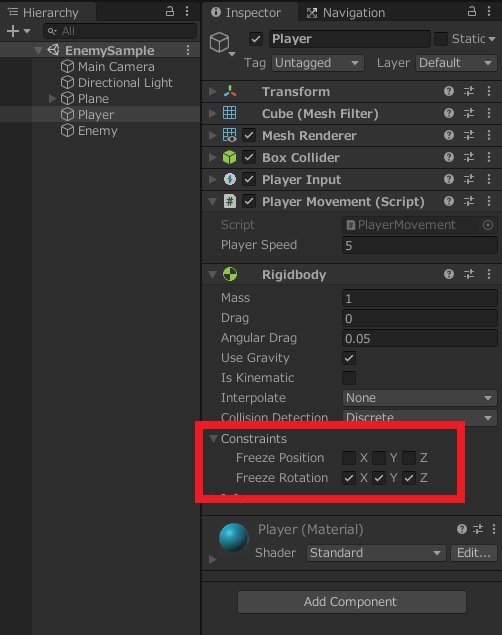
「FreezePosition」と「FreezeRotation」の2つ設定したい場合は、下記の様な感じに。
void Start() { _rigidbody = GetComponent<Rigidbody>(); _rigidbody.constraints = RigidbodyConstraints.FreezePositionY| RigidbodyConstraints.FreezeRotation; }
Rigidbodyの軸コントロールは下記のスクリプトリファレンスが参考になるかも
スクリプトや、Animatorとかも下記のような感じに追加できます。
[RequireComponent(typeof(Player))] [RequireComponent(typeof(Animator))]
エラー防止にはなるけど、エラーでしっかり出て欲しい人もいると思うので、実際に使えるのかってのは人によるのかも。